Guia: Blazor aplicativos híbridos 101

A busca pela integração perfeita entre ambientes da Web e de desktop é um dos principais desafios no desenvolvimento de aplicativos Blazor. À medida que os usuários finais exigem cada vez mais experiências multiplataforma, os desenvolvedores buscam soluções robustas capazes de reunir a flexibilidade dos aplicativos da Web com o desempenho e a funcionalidade das interfaces de desktop.
É por isso que reunimos o know-how de nossos especialistas neste whitepaper com Jun-ichi Sakamoto, Engenheiro de Consultoria Técnica da Infragistics e detentor do Microsoft MVP Award, que fornece insights práticos, práticas recomendadas e um guia técnico para todos que desejam começar a usar o Blazor Hybrid. Conheça as especificidades do desenvolvimento de aplicativos híbridos Blazor, como Blazor híbrido com .NET MAUI, híbrido Blazor com WPF Blazor híbrido com Windows Forms etc. Aprofunde-se em todas as vantagens e desvantagens, estratégias de implementação, metodologias de implantação com Ignite UI Blazor e muito mais.
O que este whitepaper abordará:
- Explicando Blazor em geral
- Introdução ao Blazor Hybrid
- Como funciona Blazor híbrido
- Prós e contras do Blazor híbrido
- As melhores práticas
- Integração com tecnologias existentes
- Usando Blazor Híbrido & Ignite UI Blazor
- Recursos adicionais
Você quer aproveitar o Blazor Hybrid para criar aplicativos que abrangem dispositivos móveis, desktop e a web, usando as ferramentas que você conhece e as habilidades que possui? Para entender como fazer isso e o que exatamente é Blazor Híbrido, daremos um passo abaixo para explicar brevemente Blazor si. E até mesmo um passo abaixo disso para ver do que Blazor parte.
Explicando Blazor antes de se aprofundar em Blazor híbrido
Blazor em si é uma plataforma de pilha completa que elimina a necessidade de chamar JavaScript complexo ao executar aplicativos da web. Em vez disso, tudo o que você escreve está em C#. Mas outra grande coisa é que Blazor desenvolvimento é baseado em componentes. Isso significa que você pode escrever pequenos componentes usando Razor sintaxe (combinando marcação HTML e código C#) e compartilhá-los em seu aplicativo ou em vários outros projetos em execução na Web.
Agora, para dar um passo atrás, Blazor também faz parte do ASP.NET Core. Que por si só é uma poderosa plataforma web que permite desenvolver aplicativos web, APIs, serviços, sites, serviços em segundo plano, etc. Tudo em C# e .NET. Reunidos, Blazor e ASP.NET Core garantem a reutilização e o compartilhamento de código entre componentes do lado do servidor e do lado do cliente, otimização de desempenho, tratamento de solicitações mais eficiente e muito mais.
Mas a flexibilidade de Blazor não termina aqui. Na verdade, ele se estende a três cenários em que Blazor trabalha: Blazor Server (com sua abordagem inovadora que dá suporte a um modo de servidor interativo e a um modo de renderização estática, permitindo que os aplicativos sejam executados no servidor sobre o tempo de execução completo do .NET) e Blazor WebAssembly (usado para criar aplicativos do lado do cliente diretamente no navegador em um tempo de execução do .NET baseado em WebAssembly). A melhor parte é que Blazor Server e Blazor WebAssembly funcionam perfeitamente bem no modo de renderização automática.
E então, há o terceiro modelo –Blazor Hybrid. Qual é o assunto principal aqui que será discutido em detalhes nas seções a seguir.
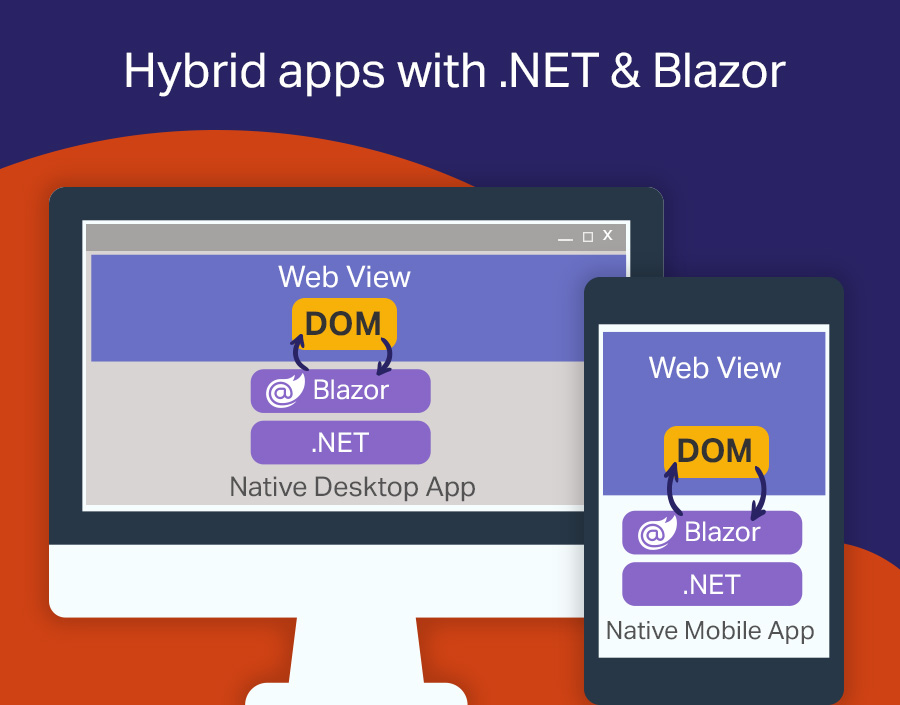
Introdução ao Blazor Hybrid: O que é Blazor híbrido?
Blazor Hybrid é um padrão de desenvolvimento da Microsoft que combina tecnologias da Web como HTML, CSS e, opcionalmente, JavaScript com estruturas nativas de desenvolvimento de aplicativos móveis ou de desktop. Em essência, um aplicativo híbrido Blazor será executado no lado do cliente e não terá acesso direto a nenhum dos recursos do servidor e da rede.
Para aplicativos de desktop, Blazor Hybrid pode implementar estruturas como:
- .NET MAUI
- WPF
- Formulários do Windows
Nesse caso, Blazor componentes são inseridos em contêineres de aplicativos nativos usando controles como Blazor WebView.
Para aplicativos móveis em execução no iOS e no Android, Blazor Híbrido pode usar o .NET MAUI.
Aqui, os componentes podem ser incorporados em interfaces de usuário móveis nativas usando componentes como o Blazor WebView.
Como funciona Blazor híbrido?
Quando você tem um aplicativo híbrido Blazor, há Razor componentes renderizados em um controle de exibição da Web inserido por meio de um canal de interoperabilidade local e eles são executados nativamente no dispositivo. O código Blazor aqui é carregado e executado rapidamente, com os componentes obtendo acesso total aos recursos nativos do dispositivo por meio da plataforma .NET. Mas vamos ver como Blazor Híbrido funciona com diferentes estruturas de aplicativos nativos do .NET.
Blazor Hybrid + .NET MAUI
Para começar, o .NET MAUI oferece uma estrutura unificada para a criação de aplicativos direcionados a várias plataformas, incluindo iOS, Android, macOS, Windows e muito mais. Uma grande vantagem é que, ao usar o .NET MAUI e Blazor juntos, os aplicativos são criados usando uma única base de código e você pode compartilhar facilmente o código e os componentes da interface do usuário entre diferentes destinos (móvel, desktop e Web). Dessa forma, você se beneficia de uma experiência de desenvolvimento consistente e reduz a necessidade de escrever código separado para cada plataforma.
Mas para que Blazor componentes sejam incorporados à interface do usuário nativa de cada plataforma, Blazor aplicativos híbridos aproveitam Blazor controle WebView. Ele funciona como um Blazor detentor de componente, permitindo que você integre Blazor componentes e a lógica da interface do usuário perfeitamente em contêineres de aplicativos nativos e aplicativos híbridos. As coisas que você pode fazer com o controle Blazor WebView são:
- Hospede Blazor componentes que podem coexistir com outros elementos nativos da interface do usuário.
- Interaja com Blazor componentes e passe dados entre eles.
- Lide com eventos como cliques ou alterações de entrada e acione ações.
- Controle coisas como inicialização, renderização, etc.
Blazor Hybrid + WPF
Outra maneira de criar aplicativos híbridos Blazor é com o WPF – uma estrutura de interface do usuário de pilha completa com ferramentas abrangentes e um ecossistema rico, permitindo que você desenvolva aplicativos de desktop ricos em recursos. Aqui, você pode usar os amplos recursos que o WPF oferece e garantir a capacidade de resposta e projetos de área de trabalho visualmente atraentes enquanto integra Blazor componentes em aplicativos novos ou existentes.
O mais interessante aqui é que ele utiliza o controle WebView2 que permite incorporar HTML, CSS e JavaScript em seus aplicativos nativos. No entanto, você também pode usar o BlazorWebView para WPF, fornecendo uma maneira simples de inserir conteúdo Blazor em aplicativos da área de trabalho do Windows.
Blazor Hybrid + Windows Forms
Blazor Hybrid também fornece BlazorWebView para essa estrutura. Essencialmente, o controle BlazorWebView atua como uma ponte entre Blazor componentes e o aplicativo Windows Forms que você está criando. É fundamental para o processo porque fornece um contêiner para hospedar Razor componentes na interface do usuário do Windows Forms, permitindo que eles sejam renderizados em um Modo de Exibição da Web inserido enquanto aproveitam todos os recursos nativos do sistema operacional Windows. Como Blazor componentes são hospedados no BlazorWebView, eles podem se comunicar com o código nativo do Windows Forms usando mecanismos de interoperabilidade. Isso permite coisas como troca de dados, manipulação de eventos e outros, tornando-o ideal para uma ampla variedade de cenários de desktop.
Prós e contras do Blazor híbrido
Até agora, você deve ter entendido como funciona Blazor Hybrid e o que é um aplicativo Blazor Hybrid. Agora, vamos passar para as vantagens e desvantagens que isso pode trazer.
Blazor Vantagens híbridas:
- Trata-se de desempenho, flexibilidade e velocidade.
- Blazor Os aplicativos híbridos são executados usando o runtime do .NET nativamente em plataformas de destino.
- Blazor Os aplicativos híbridos podem ser executados offline depois de baixados (por outro lado, os componentes renderizados para o modelo de hospedagem do Blazor Server não podem ser executados quando não há conexão com o servidor.)
- Permite descarregar a maior parte ou todo o processamento para os clientes. Dessa forma, o aplicativo processa uma quantidade significativa de dados com mais facilidade.
- Você pode usar habilidades de back-end ou .NET C# para desenvolver a interface do usuário de front-end do seu aplicativo.
- Permite acesso total aos recursos da API do cliente nativo, com Razor componentes em execução diretamente no aplicativo nativo.
- Capacidade de criar aplicativos que têm acesso a recursos e capacidades nativas da plataforma.
Blazor Desvantagens do híbrido:
- Às vezes, pode ser necessário executar código gerenciado (C#) e nativo, o que pode resultar em sobrecargas de desempenho.
- Embora Blazor aplicativos híbridos sejam compilados em um ou mais ativos de implantação independentes, os ativos geralmente são fornecidos aos clientes por meio de uma loja de aplicativos de terceiros.
- Blazor Os aplicativos híbridos exigem um instalador e um mecanismo de implantação específico da plataforma.
- Pode haver limitações em termos de recursos e APIs específicos da plataforma.
- Talvez seja necessário investir mais tempo para aprender como integrá Blazor adequadamente à plataforma de destino.
- Quando há limitações ou problemas com o controle WebView, isso pode comprometer a funcionalidade e o desempenho de todo o aplicativo.
Práticas recomendadas ao criar aplicativos com Blazor híbrido
Componentes
Evite criar componentes monolíticos enormes. Em vez disso, tente combinar componentes pequenos e concisos para criar componentes maiores que possam lidar com casos mais complexos. Além disso, nos componentes, esforce-se para se concentrar na visualização do modelo para exibições e na interação com as entradas do usuário. Considere delegar o processamento específico do domínio a um serviço externo. Isso aumentará a capacidade de manutenção e simplificará a implementação de testes automatizados. Se você planeja lançar o aplicativo híbrido não apenas como um aplicativo móvel ou de desktop, mas também como um aplicativo Web, isole Razor componentes e classes compartilhadas em um projeto de biblioteca de classes Razor e faça referência a ele no projeto MAUI.
Vinculação de dados
Deve ser melhor que a interação entre os componentes seja feita por vinculação de dados. Os designs que usam as diretivas "@ref" para obter a referência de um componente e, em seguida, usam as propriedades e os métodos desse componente são desencorajados, exceto em alguns cenários, como componentes de caixa de diálogo. Por exemplo, se você quiser fazer uma opção específica inicialmente selecionada em uma lista suspensa, não chame o método de seleção da lista suspensa. Em vez disso, implemente uma variável de campo que indique a opção selecionada e vincule essa variável de campo à lista suspensa.
Roteamento
Se você também estiver lançando-o como um aplicativo Web, considere criar um roteamento de URL que use diretivas "@page". Por exemplo, se você estiver criando uma página para pesquisar uma listagem, inclua as palavras-chave de pesquisa na cadeia de caracteres de consulta da URL para permitir cenários de compartilhamento de links.
Validação de formulário
Recomenda-se que a validação de formulário seja implementada com base em "EditForm" e "EditorContext" do Blazor. Blazor fornece um mecanismo de validação de formulário declarativo usando o componente "DataAnnotaionValidator", com base em atributos que anotam o modelo, por isso é bom usá-lo. Como alternativa, várias outras bibliotecas de validação de formulários de terceiros também estão disponíveis.
Dependências
As classes de serviço individuais devem ser simplificadas sob o princípio da responsabilidade única. No entanto, isso exigirá que muitas classes de serviço trabalhem juntas. Nesse caso, é recomendável que os contêineres "DI (injeção de dependência)" sejam utilizados ativamente para resolver dependências entre serviços. Delegar a responsabilidade de gerar objetos de serviço ao contêiner de DI aumentará enormemente a capacidade de manutenção e a produtividade.
Além disso, ao usar a funcionalidade nativa da plataforma, você deve abstrair a funcionalidade em interfaces, implementar classes concretas que implementam essa interface para cada plataforma e injetá-las e usá-las em componentes por meio do contêiner de DI. A injeção de dependência não serve apenas para conectar simulações em testes de unidade.
Gerenciamento de estado
O estado da interface do usuário específico do componente, como o estado aberto/fechado de um componente sanfonado, deve ser gerenciado por meio de variáveis ou propriedades de campo de componente. Se o aplicativo também for publicado como um aplicativo Web, considere usar parâmetros de consulta de URL para salvar o estado da interface do usuário. Falando sobre gerenciamento de estado global, como compartilhar temas de esquema de cores em todos os componentes da interface do usuário, o uso de parâmetros em cascata será útil. O modelo de nível de domínio será melhor tratado por meio da injeção de dependência, tornando-o uma classe de serviço. É claro que existem várias outras bibliotecas de gerenciamento de estado global que você pode considerar usar.
Autenticação e autorização
Não implemente autenticação e autorização por conta própria. Em vez disso, use bibliotecas confiáveis existentes. Para a infraestrutura de autenticação, considere usar um serviço chamado "ID como serviço" em vez de depender de sua própria implementação. Ao implementar a autorização usando o protocolo OAuth para integração com serviços Web externos, especialmente para aplicativos de desktop ou móveis, certifique-se de evitar que os códigos de autorização sejam roubados devido ao registro duplicado de esquemas personalizados, como o uso de PKCE.
Testes automatizados
Mesmo para o desenvolvimento de aplicativos híbridos, o teste automatizado é essencial para o desenvolvimento sustentável de software e o crescimento do produto. Isolar o processamento específico do domínio em classes de serviço torna os testes automatizados mais fáceis de implementar e manter. Os testes de unidade também podem ser implementados para o domínio da interface do usuário usando bibliotecas de terceiros, como o bUnit. Se o aplicativo também for lançado como um aplicativo da Web, o teste de ponta a ponta também pode ser realizado com base no conhecimento do desenvolvimento de aplicativos da Web usando ferramentas de terceiros, como o Playwright.
Integrando Blazor híbrido com tecnologias existentes
Integração com APIs ASP.NET Core
ASP.NET Core é a mesma plataforma .NET que o aplicativo Blazor Hybrid, portanto, a combinação desses aplicativos nos permite desenvolver aplicativos fortemente tipados, seguros e eficientes, como o compartilhamento de classes de transferência de dados. A comunicação bidirecional em tempo real baseada em WebSocket (conhecida como SignalR) ou gRPC pode ser facilmente implementada, bem como a API REST.
Integração com JavaScript
Por meio do recurso de interoperabilidade JavaScript do Blazor, as implementações de interface do usuário que são difíceis de alcançar apenas com Blazor podem ser realizadas usando JavaScript. Felizmente, os desenvolvedores não precisam necessariamente escrever JavaScript por conta própria, que já pode ser distribuído como uma biblioteca de terceiros como um pacote NuGet. Também é possível usar bibliotecas JavaScript conhecidas no WebView para trabalhar em conjunto com Blazor
Integração com Blazor WebAssembly
Se o aplicativo também for lançado como um aplicativo Web, ele poderá ser lançado como um PWA e funcionar offline se puder ser lançado no modelo de aplicativo Blazor WebAssembly.
Como usar Blazor híbrido com Ignite UI Blazor
Ignite UI é uma biblioteca completa de centenas de componentes de interface do usuário para todas as principais estruturas da web. Ele inclui as grades mais rápidas do mercado, gráficos de alto desempenho otimizados para velocidade e desempenho e qualquer outro controle necessário para a experiência de criação de aplicativos mais abrangente. Quando se trata de Ignite UI for Blazor em particular, você tem o poder de criar aplicativos da Web ricos em recursos usando um conjunto rápido de componentes e recursos. Isso inclui Grade de Dados, Grade Dinâmica, Grade Hierárquica e muito mais; qualquer gráfico que você precisar – Área, Pizza, Financeiro, etc., além de controles exclusivos como Stepper, Dock Manager e muitos outros.
Introdução ao Ignite UI e Blazor híbrido
Nesta seção, mostraremos como criar e executar um aplicativo híbrido do Blazor com o .NET MAUI e Ignite UI for Blazor e mostraremos um exemplo Blazor híbrido concluído no final. Lembre-se de que Blazor aplicativo híbrido -MAUI pode ser desenvolvido em distribuições Windows, macOS ou Linux. No entanto, para os fins deste artigo, descreveremos apenas um cenário baseado no Windows.
Pré-requisitos:
- SDK do .NET 8 ou superior
- A versão mais recente do Visual Studio 2022 ou superior, com as seguintes cargas de trabalho:
- Desenvolvimento da interface do usuário do aplicativo multiplataforma .NET
- ASP.NET e Desenvolvimento Web
Passo 1: Crie um novo projeto ".NET MAUI Blazor Hybrid App" no Visual Studio.
Passo 2: Forneça um nome e um local do projeto e, depois de fazer isso, clique em Criar.
Etapa 3: No Visual Studio, abra o gerenciador de pacotes NuGet selecionando Project → Gerenciar Pacotes NuGet. Pesquise e instale o pacote NuGet IgniteUI.Blazor.
Passo 4: Registe-se Ignite UI for Blazor
Primeiro, você terá que abrir o arquivo MauiProgram.cs e registrar o Ignite UI for Blazor Service chamando builder. Services.AddIgniteUIBlazor. Em seguida, adicione o IgniteUI. Blazor. Controla o namespace no arquivo_Imports.razor.
@using IgniteUI.Blazor.Controls
Quando estiver pronto, adicione a Folha de Estilo no elemento<head> do arquivo wwwroot/index.html:
<head> <link rel="stylesheet" href="_content/IgniteUI.Blazor/themes/light/bootstrap.css" /> </head>
Para continuar e adicionar a Referência de script ao arquivo wwwroot/index.html, faça o seguinte:
<script src="_content/IgniteUI.Blazor/app.bundle.js"></script> <script src="_framework/blazor.webview.js" autostart="false"></script>
Passo 5: Adicionar componentes Ignite UI for Blazor
É assim que se faz:
<IgbCard style="width:350px"> <IgbCardMedia> <img src="https://images.unsplash.com/photo-1541516160071-4bb0c5af65ba?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=350&q=80" /> </IgbCardMedia> <IgbCardHeader> <h4>Jane Doe</h4> <h6>Professional Photographer</h6> </IgbCardHeader> <IgbCardContent>Hi! I'm Jane, photographer and filmmaker. Photography is a way of feeling, of touching, of loving. What you have caught on film is captured forever... it remembers little things, long after you have forgotten everything.</IgbCardContent> <IgbCardActions> <IgbButton>More Info</IgbButton> </IgbCardActions> </IgbCard>
E é assim que você cria um aplicativo .NET MAUI Blazor para Windows.
Recursos Blazor úteis adicionais
Com o Blazor se tornando uma das estruturas mais populares e amplamente utilizadas para o desenvolvimento de aplicativos da Web modernos, é essencial saber ir além do básico. É por isso que você pode explorar os guias adicionais listados abaixo, para que possa aprofundar sua compreensão e aprimorar sua experiência em desenvolvimento Blazor. Aprofunde-se em tópicos avançados, domine as práticas recomendadas e mantenha-se atualizado.
Continue lendo
Preencha o formulário para continuar lendo.